Course overview
This course covers the language features in C# and the .NET platform. You’ll get a deep and broad understanding of C# as a programming language and gain confidence to explore the richness of the .NET Framework library including asynchronous programming and LINQ.
Duration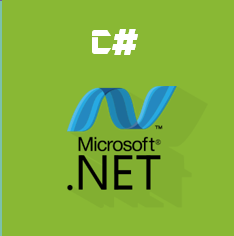
4 Days
Prerequisites
- At least 6 months programming experience
- Familiarity with OO concepts would be an advantage
What you’ll learn
- Essential C# syntax
- Implementing object-oriented designs in C#
- Using generics, collection classes, and exceptions
- What’s new in C# and .NET
- Using LINQ
- Introduction to asynchronous programming
Course details
Introduction to C# and .NET
- .NET Framework building blocks
- Hello world in C#
- Using Visual Studio
- Namespaces and assemblies
Core C# Programming Constructs
- Variables, operators, and statements
- Reference types vs. value types
- Conversions
- Nullable types
- Using the Console and String classes
- Flow-of-control
- C# pattern matching
- Using implicit types
More C# Programming Constructs
- Defining methods
- Input, output, and optional parameters
- C# local functions
- C# enhancements for out and ref
- Method overloading
- Arrays
- Structures
Defining and Using Classes
- Defining classes
- Defining constructors and finalizers
- Defining properties
- Creating and disposing objects
- Defining constants and read-only fields
- Static members
- Partial classes/methods
Inheritance and Polymorphism
- Defining base classes and derived classes
- Overriding methods
- Abstract classes
- Interfaces
Exception Handling
- Defining Try/Catch/Finally blocks
- Throwing exceptions
- C#throw expressions
- Defining new exception classes
- Defining Using blocks
Creating Collections of Objects
- Overview of generics
- Using List collections
- Using Dictionary collections
What’s New in C#
- Static using syntax
- Auto-property initializers
- Dictionary initializers
- Exception filters
- String interpolation
Delegates, Events, and Lambdas
- Overview
- Defining simple delegates
- Defining and handling events
- Using lambda expressions
- Expression-bodied members in C# 6 and C# 7
Additional Language Features
- Operator overloading
- Extension methods
- Object initializers
- Anonymous types
- C# 7 tuples
Introduction to LINQ
- The role of LINQ
- Simple LINQ query expressions
- Using LINQ with collections
- LINQ query operators
Introduction to Asynchronous Programming
- Understanding the async and await keywords
- C#7 generalized async returns
- Implementing asynchronous code